I just created a little html game using just plain JavaScript. I don't really want to play the game, so I though why not pass it on, maybe some reader will enjoy it or put it on their own website.
Please note that this may not be the best way to program the game. I am sure that their are shortcuts to iterate more effectively through things. This was basically just a result of to much coffee and not sleeping rather than a serious effort to create a clean game.
So if you are a JavaScript master, and would like to clean it up, please feel free, and send it back to me.
Also note that it was done for Firefox, and though I think for the most part I have stuck with cross browser compatible JavaScript, if I haven't done it fully, let me know and some other non sleeping night I will clean it up a bit.
BTW this is by far the most effective way to learn how to use a computer language (perhaps even a regular language)...play with it. Think of programming/scripting as lego blocks for your computer.
Anyway, Enjoy ;~)
NOTE, since posting it I noticed there are still some errors. If you are keen, see if you can find them. I also uploaded the game to a website just for kicks - http://www.sandcurves.com/tictactoe.htm
<html><head><title>Tic-Tac-Toe</title>
<style type="text/css">
body{
margin:50px 200px 0 200px;
background-color:gray;
}
h1{
color:white;
font-size:200%;
}
p{
color:#cccccc;
font-size:200%;
font-weight:700;
}
input{
background-color:#dddddd;
border:none;
width:inherit;
text-align:center;
font-size:400%;
color:white;
font-weight:800;
}
input[type=reset]{
background-color:gray;
border:1px solid #cccccc;
font-size:150%;
cursor:pointer;
margin-top:50px;
padding:7px;
}
td{
text-align:center;
background-color:#dddddd;
border:gray 4px solid;
width:100px;
height:100px;
}
</style>
<script type="text/javascript">
var playeR = "First";
var zed = 0;
function startOff(){
document.getElementById('whichplayer').innerHTML = playeR;
document.minIn.t1v.value = "";
document.minIn.t2v.value = "";
document.minIn.t3v.value = "";
document.minIn.m1v.value = "";
document.minIn.m2v.value = "";
document.minIn.m3v.value = "";
document.minIn.b1v.value = "";
document.minIn.b2v.value = "";
document.minIn.b3v.value = "";
}
function nextMove(a){
if (playeR == "First"){
playeR = "Second";
var marK = "X";
}
else {
playeR = "First";
var marK = "O";
}
document.getElementById('whichplayer').innerHTML = playeR;
switch (a){
case ('t1'):document.minIn.t1v.value = marK;break;
case ('t2'):document.minIn.t2v.value = marK;break;
case ('t3'):document.minIn.t3v.value = marK;break;
case ('m1'):document.minIn.m1v.value = marK;break;
case ('m2'):document.minIn.m2v.value = marK;break;
case ('m3'):document.minIn.m3v.value = marK;break;
case ('b1'):document.minIn.b1v.value = marK;break;
case ('b2'):document.minIn.b2v.value = marK;break;
case ('b3'):document.minIn.b3v.value = marK;break;
}
var checka = document.minIn.t1v.value;
var checkb = document.minIn.t2v.value;
var checkc = document.minIn.t3v.value;
var checkd = document.minIn.m1v.value;
var checke = document.minIn.m2v.value;
var checkf = document.minIn.m3v.value;
var checkg = document.minIn.b1v.value;
var checkh = document.minIn.b2v.value;
var checki = document.minIn.b3v.value;
if((checka == checkb) && (checkb == checkc)){
if (checka != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checkd == checke) && (checke == checkf)){
if (checkd != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checkg == checkh) && (checkh == checki)){
if (checkg != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checka == checkd) && (checkd == checkg)){
if (checka != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checkb == checke) && (checke == checkh)){
if (checkb != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checkc == checkf) && (checkf == checki)){
if (checkc != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checka == checke) && (checke == checki)){
if (checka != "" || 0){zed = 1;}
else{ zed = 0; }
}
else if((checkc == checke) && (checke == checkg)){
if (checkc != "" || 0){zed = 1;}
else{ zed = 0; }
}
else{
zed = 0;
}
if (zed == 1){
if (marK == "X"){
alert("First player wins");
}
else {
alert("Second player wins");
}
}
}
</script>
</head>
<body onload="startOff()">
<h1>Tic-Tac-Toe</h1>
<p><span id="whichplayer"></span> player</p>
<form name="minIn">
<table>
<tr>
<td id="t1"><input type="text" name="t1v" onclick="nextMove('t1')" /></td>
<td id="t2"><input type="text" name="t2v" onclick="nextMove('t2')" /></td>
<td id="t3"><input type="text" name="t3v" onclick="nextMove('t3')" /></td>
</tr>
<tr>
<td id="m1"><input type="text" name="m1v" onclick="nextMove('m1')" /></td>
<td id="m2"><input type="text" name="m2v" onclick="nextMove('m2')" /></td>
<td id="m3"><input type="text" name="m3v" onclick="nextMove('m3')" /></td>
</tr>
<tr>
<td id="b1"><input type="text" name="b1v" onclick="nextMove('b1')" /></td>
<td id="b2"><input type="text" name="b2v" onclick="nextMove('b2')" /></td>
<td id="b3"><input type="text" name="b3v" onclick="nextMove('b3')" /></td>
</tr>
</table>
<input type="reset" value="CLEAR GAME" />
</form>
</body>
</html>
Tuesday, October 20, 2009
Tic-TacToe game with JavaScript
Posted by
Vernon
at
11:50 PM
Tic-TacToe game with JavaScript
2009-10-20T23:50:00-07:00
Vernon
game|javascript|tic-tac-toe|
Comments

Labels:
game,
javascript,
tic-tac-toe
Wednesday, October 14, 2009
Number to Ascii Values
Hey, I thought I would just post these values. I know it's rather easy to find out, but often you need to use some of these symbols in your work, so here they are. I was just playing around with php and created a script that would print them out. I'll give the script at the end.
Number Ascii Value
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 !
34 "
35 #
36 $
37 %
38 &
39 '
40 (
41 )
42 *
43 +
44 ,
45 -
46 .
47 /
48 0
49 1
50 2
51 3
52 4
53 5
54 6
55 7
56 8
57 9
58 :
59 ;
60 <
61 =
62 >
63 ?
64 @
65 A
66 B
67 C
68 D
69 E
70 F
71 G
72 H
73 I
74 J
75 K
76 L
77 M
78 N
79 O
80 P
81 Q
82 R
83 S
84 T
85 U
86 V
87 W
88 X
89 Y
90 Z
91 [
92 \
93 ]
94 ^
95 _
96 `
97 a
98 b
99 c
100 d
101 e
102 f
103 g
104 h
105 i
106 j
107 k
108 l
109 m
110 n
111 o
112 p
113 q
114 r
115 s
116 t
117 u
118 v
119 w
120 x
121 y
122 z
123 {
124 |
125 }
126 ~
127
128 €
129
130 ‚
131 ƒ
132 „
133 …
134 †
135 ‡
136 ˆ
137 ‰
138 Š
139 ‹
140 Œ
141
142 Ž
143
144
145 ‘
146 ’
147 “
148 ”
149 •
150 –
151 —
152 ˜
153 ™
154 š
155 ›
156 œ
157
158 ž
159 Ÿ
160
161 ¡
162 ¢
163 £
164 ¤
165 ¥
166 ¦
167 §
168 ¨
169 ©
170 ª
171 «
172 ¬
173
174 ®
175 ¯
176 °
177 ±
178 ²
179 ³
180 ´
181 µ
182 ¶
183 ·
184 ¸
185 ¹
186 º
187 »
188 ¼
189 ½
190 ¾
191 ¿
192 À
193 Á
194 Â
195 Ã
196 Ä
197 Å
198 Æ
199 Ç
200 È
201 É
202 Ê
203 Ë
204 Ì
205 Í
206 Î
207 Ï
208 Ð
209 Ñ
210 Ò
211 Ó
212 Ô
213 Õ
214 Ö
215 ×
216 Ø
217 Ù
218 Ú
219 Û
220 Ü
221 Ý
222 Þ
223 ß
224 à
225 á
226 â
227 ã
228 ä
229 å
230 æ
231 ç
232 è
233 é
234 ê
235 ë
236 ì
237 í
238 î
239 ï
240 ð
241 ñ
242 ò
243 ó
244 ô
245 õ
246 ö
247 ÷
248 ø
249 ù
250 ú
251 û
252 ü
253 ý
254 þ
255 ÿ
Very easy php script for generating a table withe the ascii values.
The magic is in the chr() function. If you know php you would know that you could jump out of the php and just draw the table in html, but that seems like more work in this situation.
If you don't know php, you do need a server installed to run it. I will cover some of that stuff later in this blog. For now, just a little script for kicks.
<?php
print "<table><tr><th>Number</th><th>Ascii Value</th></tr>";
for ($x=0;$x<256;$x++){
print "<tr>";
$valAscii = chr($x);
print "<td>$x</td><td>$valAscii</td>";
print "</tr>";
}
print "</table>";
?>
Number Ascii Value
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 !
34 "
35 #
36 $
37 %
38 &
39 '
40 (
41 )
42 *
43 +
44 ,
45 -
46 .
47 /
48 0
49 1
50 2
51 3
52 4
53 5
54 6
55 7
56 8
57 9
58 :
59 ;
60 <
61 =
62 >
63 ?
64 @
65 A
66 B
67 C
68 D
69 E
70 F
71 G
72 H
73 I
74 J
75 K
76 L
77 M
78 N
79 O
80 P
81 Q
82 R
83 S
84 T
85 U
86 V
87 W
88 X
89 Y
90 Z
91 [
92 \
93 ]
94 ^
95 _
96 `
97 a
98 b
99 c
100 d
101 e
102 f
103 g
104 h
105 i
106 j
107 k
108 l
109 m
110 n
111 o
112 p
113 q
114 r
115 s
116 t
117 u
118 v
119 w
120 x
121 y
122 z
123 {
124 |
125 }
126 ~
127
128 €
129
130 ‚
131 ƒ
132 „
133 …
134 †
135 ‡
136 ˆ
137 ‰
138 Š
139 ‹
140 Œ
141
142 Ž
143
144
145 ‘
146 ’
147 “
148 ”
149 •
150 –
151 —
152 ˜
153 ™
154 š
155 ›
156 œ
157
158 ž
159 Ÿ
160
161 ¡
162 ¢
163 £
164 ¤
165 ¥
166 ¦
167 §
168 ¨
169 ©
170 ª
171 «
172 ¬
173
174 ®
175 ¯
176 °
177 ±
178 ²
179 ³
180 ´
181 µ
182 ¶
183 ·
184 ¸
185 ¹
186 º
187 »
188 ¼
189 ½
190 ¾
191 ¿
192 À
193 Á
194 Â
195 Ã
196 Ä
197 Å
198 Æ
199 Ç
200 È
201 É
202 Ê
203 Ë
204 Ì
205 Í
206 Î
207 Ï
208 Ð
209 Ñ
210 Ò
211 Ó
212 Ô
213 Õ
214 Ö
215 ×
216 Ø
217 Ù
218 Ú
219 Û
220 Ü
221 Ý
222 Þ
223 ß
224 à
225 á
226 â
227 ã
228 ä
229 å
230 æ
231 ç
232 è
233 é
234 ê
235 ë
236 ì
237 í
238 î
239 ï
240 ð
241 ñ
242 ò
243 ó
244 ô
245 õ
246 ö
247 ÷
248 ø
249 ù
250 ú
251 û
252 ü
253 ý
254 þ
255 ÿ
Very easy php script for generating a table withe the ascii values.
The magic is in the chr() function. If you know php you would know that you could jump out of the php and just draw the table in html, but that seems like more work in this situation.
If you don't know php, you do need a server installed to run it. I will cover some of that stuff later in this blog. For now, just a little script for kicks.
<?php
print "<table><tr><th>Number</th><th>Ascii Value</th></tr>";
for ($x=0;$x<256;$x++){
print "<tr>";
$valAscii = chr($x);
print "<td>$x</td><td>$valAscii</td>";
print "</tr>";
}
print "</table>";
?>
Posted by
Vernon
at
8:08 AM
Number to Ascii Values
2009-10-14T08:08:00-07:00
Vernon
Ascii values by number|
Comments

Labels:
Ascii values by number
Friday, October 9, 2009
Tutorial and Guidelines for creating a custom Twitter Background
This post is a reply to a request from a good friend of mine Shem for help on designing a custom Twitter Background. As a serious professional wildlife photographer, Shem is interested in creating a very professional twitter background to reflect his work.
Why should you bother with a customized background image for twitter? Don't most people manage their twitter accounts with programs like TweetDeck and so on? Yes, but still, if someone clicks your "Follow Me on Twitter" link, they will land on that page. So you want to make a good impression if you want followers.
This guideline is a simple step by step way of doing it. Note, I am doing this on Ubuntu Linux and the tools I use may not be what you have, but none of them are hard to get by, basically I just use a browser, a screenshot thingi (to take a picture of your screen), and a graphics editor.
Before we get started, have a look at some of the amazing twitter backgrounds that people have produced. I will show you a step by step way of doing it, but I can't tell you how to make it artistically outstanding, that's up to you. Take a look at this link: http://twitterbackgroundsgallery.com/
1. Open your twitter account as it is now.
2. Okay, the biggest challenge with your twitter background is the fact that it sits behind your main twitter screen, and only overlaps on the sides. This means that you want your most improtant information to stick out of the sides. In order to make sure that even people with really small screens are getting this, first resize your screen to about 1024 width and 768 height. If you don't know how to do this, there is one rather simple way, just use Javascript.
Hit enter and you should have a really small screen.
3. Now use a screenshot tool to take a screenshot of your twitter page in this small resolution.
4. Open your favorite Graphics Editor program. You want to make sure that the program you use allows you to use layers, so most lightweight programs will not do. I use Gimp, which is a very good open source graphics editor. If you don't have Adobe Photoshop or something in that league, I recommend that you download Gimp
5. I am going to carry on with the explanation in Gimp, but I am sure that you will figure out how to do it in your own program. Create a new image. It doesn't matter if you set the background to white or transparent. Create a picture that is 1280px times 1024px (px being Pixils)
6. Now comes the more tricky part. You need to go 'open as layer' or whatever the equivalent is in photoshop, and select the screenshot of your twitter account on the smaller screen, and open it.
7. Use the 'align' tool to set the twitter image to the top left side. It should look something like the image below.
8. Okay, the only real reason for having the upper image is to show us what area we have for our text. So, staying in this layer, use a 'rectangular select' tool and select the area on the left of the main twitter content. This is where you want to write your stuff.
I am not sure how clear this screen shot is to you, but the area left of the white is selected as a rectangular section. You can see that I didn't select right up to the top, that's your choice. But basically this is the area that even on a small screen is not going to get run over by the main twitter content.
9. Create a new layer. In gimp you will still maintain the selection in the new layer.
10. Now, you probably don't want to maintain the selected area, so a simple thing to do is to simply go "Select" and click "invert selection" Now use your fill tool and color the remaining area into one solid color. This color doesn't matter...it's not going to be your background. It is simply there to show you the remaining space that you have to work with.
11. Go to your "layers" dialog box and make the old twitter layer invisible.
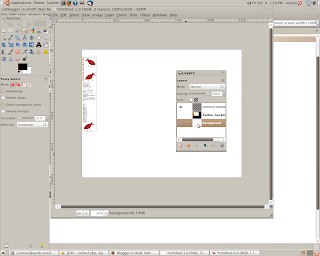
15. With this 'background' layer selected you can either put an image in there or simply create a fill with colors. Anything you want. Take a two things into consideration
a. Does it not wash out the dialog created in the other layer.
b. Make sure that it is an image that doesn't matter if a little of it is chopped off, because of the way that gimp resizes on different size screens. Blue sky, open water, or stuff like that. Color and texture.
16. Now it really depends on how your system works, but the best way to do it is to select the layer that had the origional picture you took of your Twitter page and, once you have selected it, go to "layers" and press 'delete layer'.
17. Now click on the top layer again, and go to 'layers' again and press 'merge down' until your whole image is flattened. This is not always necicary, but remember you want this to be on the internet, so you dont' want to keep to much data in the image (in other words, make is smaller in file size).
18. Now Twitter is only going to give you an image size of 800k. You may need to play around a bit to get that right. On Gimp you can go to the Image properties and check it out.
19. Save As, choose your name and file type. I think that with jpg you get the file size you need more easily.
20. Go back to your twitter account. Click on "Settings" and then "Design" Go down to the bottom of that page and click the "Change Background Image"
21. Easy from here. Click 'Browse', find the image on your hard drive and click ''save changes".
22. Now have a look at what it looks like when it uploads. Can you read the text, how does it scrole. Go back an make changes and upload again until you are happy.
23. Go to the 'change colors' settings and change the colors as needed to match your background. You may want to copy and paste the html color values from Gimp in order to get the color values spot on.
[You can see what I came up with after playing around a bit. I used a picture of an old dune. I played around with text sizes and colors until I got something people can read when they hit on my page.
Well, thats how I had done it. Anyone with tips or ideas to share, please feel free. I hope it works for you.
If you are looking for similar tutorials, you may be interested in one of these:
Hide Your Blogger/Blogspot Navbar - Fun blogger hack
Auto-update Your Websites Copyright Dates Each Year - Be a pro
Create And Add A Favicon To Your Website - Easy fun way to improve your website's look
Make Your Main Body Wider In Blogger - With increasing use of wider screens for computers, you may want more space to add your main blog content
Why should you bother with a customized background image for twitter? Don't most people manage their twitter accounts with programs like TweetDeck and so on? Yes, but still, if someone clicks your "Follow Me on Twitter" link, they will land on that page. So you want to make a good impression if you want followers.
[By the way, I just drew this little Twitter bird on Gimp. I think it's an okayish Twitter bird. If you want to use it, go ahead, just give me a link from your blog, follow me on Twitter, or buy me a cup of coffee one day. I believe it is a African Scops Owl crossed with a cross between an Angolan Pitta and Crimson Breasted Shrike. I see those all those time :) ]
[Okay, sorry for the distraction, lets get back to the serious busyness of producing a fine Twitter Background Image]
This guideline is a simple step by step way of doing it. Note, I am doing this on Ubuntu Linux and the tools I use may not be what you have, but none of them are hard to get by, basically I just use a browser, a screenshot thingi (to take a picture of your screen), and a graphics editor.
Before we get started, have a look at some of the amazing twitter backgrounds that people have produced. I will show you a step by step way of doing it, but I can't tell you how to make it artistically outstanding, that's up to you. Take a look at this link: http://twitterbackgroundsgallery.com/
1. Open your twitter account as it is now.
2. Okay, the biggest challenge with your twitter background is the fact that it sits behind your main twitter screen, and only overlaps on the sides. This means that you want your most improtant information to stick out of the sides. In order to make sure that even people with really small screens are getting this, first resize your screen to about 1024 width and 768 height. If you don't know how to do this, there is one rather simple way, just use Javascript.
Here is the majic: Just type this little line in your navigation bar at the top of your screen: javascript:window.resizeTo(1024,768)
Hit enter and you should have a really small screen.
3. Now use a screenshot tool to take a screenshot of your twitter page in this small resolution.
4. Open your favorite Graphics Editor program. You want to make sure that the program you use allows you to use layers, so most lightweight programs will not do. I use Gimp, which is a very good open source graphics editor. If you don't have Adobe Photoshop or something in that league, I recommend that you download Gimp
5. I am going to carry on with the explanation in Gimp, but I am sure that you will figure out how to do it in your own program. Create a new image. It doesn't matter if you set the background to white or transparent. Create a picture that is 1280px times 1024px (px being Pixils)
6. Now comes the more tricky part. You need to go 'open as layer' or whatever the equivalent is in photoshop, and select the screenshot of your twitter account on the smaller screen, and open it.
7. Use the 'align' tool to set the twitter image to the top left side. It should look something like the image below.
8. Okay, the only real reason for having the upper image is to show us what area we have for our text. So, staying in this layer, use a 'rectangular select' tool and select the area on the left of the main twitter content. This is where you want to write your stuff.
9. Create a new layer. In gimp you will still maintain the selection in the new layer.
10. Now, you probably don't want to maintain the selected area, so a simple thing to do is to simply go "Select" and click "invert selection" Now use your fill tool and color the remaining area into one solid color. This color doesn't matter...it's not going to be your background. It is simply there to show you the remaining space that you have to work with.
11. Go to your "layers" dialog box and make the old twitter layer invisible.
12. Now you can work in the white layer doing whatever you want. You may want your name, blog url, a bit about yourself or just some pictures. Be creative. Keep in mind roughly how big that space is going to be after you are done. Also keep in mind that you don't want to fill the background, because you will still be putting a picture or a background fill behind it.
[More bird drawing inspiration, and this time it really does have Angolan Pitta in it somewhere.]
13. Once you have it as you want it on that space, take the fuzzy select tool and select the black space. Press delete.
14. Now go on the 'layers' dialog box and select the original background layer.
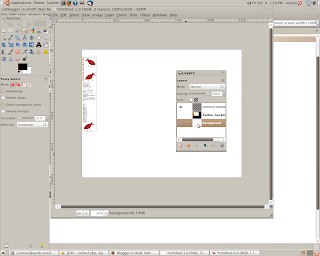
15. With this 'background' layer selected you can either put an image in there or simply create a fill with colors. Anything you want. Take a two things into consideration
a. Does it not wash out the dialog created in the other layer.
b. Make sure that it is an image that doesn't matter if a little of it is chopped off, because of the way that gimp resizes on different size screens. Blue sky, open water, or stuff like that. Color and texture.
16. Now it really depends on how your system works, but the best way to do it is to select the layer that had the origional picture you took of your Twitter page and, once you have selected it, go to "layers" and press 'delete layer'.
17. Now click on the top layer again, and go to 'layers' again and press 'merge down' until your whole image is flattened. This is not always necicary, but remember you want this to be on the internet, so you dont' want to keep to much data in the image (in other words, make is smaller in file size).
18. Now Twitter is only going to give you an image size of 800k. You may need to play around a bit to get that right. On Gimp you can go to the Image properties and check it out.
19. Save As, choose your name and file type. I think that with jpg you get the file size you need more easily.
20. Go back to your twitter account. Click on "Settings" and then "Design" Go down to the bottom of that page and click the "Change Background Image"
21. Easy from here. Click 'Browse', find the image on your hard drive and click ''save changes".
22. Now have a look at what it looks like when it uploads. Can you read the text, how does it scrole. Go back an make changes and upload again until you are happy.
23. Go to the 'change colors' settings and change the colors as needed to match your background. You may want to copy and paste the html color values from Gimp in order to get the color values spot on.
[You can see what I came up with after playing around a bit. I used a picture of an old dune. I played around with text sizes and colors until I got something people can read when they hit on my page.
Well, thats how I had done it. Anyone with tips or ideas to share, please feel free. I hope it works for you.
If you are looking for similar tutorials, you may be interested in one of these:
Hide Your Blogger/Blogspot Navbar - Fun blogger hack
Auto-update Your Websites Copyright Dates Each Year - Be a pro
Create And Add A Favicon To Your Website - Easy fun way to improve your website's look
Make Your Main Body Wider In Blogger - With increasing use of wider screens for computers, you may want more space to add your main blog content
Posted by
Vernon
at
7:20 AM
Tutorial and Guidelines for creating a custom Twitter Background
2009-10-09T07:20:00-07:00
Vernon
Gimp|Gimp Tutorial|Twitter|Twitter background design|
Comments

Labels:
Gimp,
Gimp Tutorial,
Twitter,
Twitter background design
Thursday, October 8, 2009
Auto Update Your WebsiteS Copyright Notice Each Year With PHP
If you are like me the years do sometimes just slip by. Visit family you don't see often and you know what I am talking about...the little toddlers and teenagers, that kind of stuff.
But this isn't about philosophy or self help. We have a much easier problem to fix. The dates on your copyright at the bottom of your page.
All it is going to take is just a super simple little script, but in my opinion it is useful and makes a real difference in how professional a website appears to be.
I am sure you see it often. Small business or personal home pages, very often, but sometimes you even find the website of quiet large companies with outdated copyright notices. - Example 1 - and - Example 2 -
Okay, if you have php installed on your server, this is going to be ridiculously easy, but so useful down the line. I will just cover this in a quick manner:
First off, whatever your html page, you need to save the page as a .php page instead of html. If you have links to the page already, make sure that you update them to .php instead of .html or .htm.
Changing that does nothing else to your page as it is already, it just means that you can now embed the php scripts into the page.
Okay, here is the script -
You can simply copy andpaste this into your page where you need it, but I will explain it below:
1. the <?php opens the php code and the ?> closes it. Whatever you write in there is php script.
2. We get the year from the date() function, and by putting the capital 'Y' all we get is the year. So that assigns the current year to the variable called $thisYr. Note that capitalization is important and shouldn't be changed, otherwise you will have trouble with the script.
3. The 'if {} else {}' code blocks are easy to understand. If it is this year, print the one thing, if it isn't print the other. You can change the date to whatever your beginning year is for the copyright.
4. The '©' bit is written like this, but the html page will render it like this '©' It is just the unicode symbols for the little copyright icon.
And that's basically it. You can extend it a little by putting hypertext to the copyright to a link that defines your copyright terms, such as, perhaps, a use and share and backlink or something like that.
If you are creative enough, you may think of other uses for such a simple script. It is one of the wonderful things about these types of scripting languages...small bits of code do really cool things.
But this isn't about philosophy or self help. We have a much easier problem to fix. The dates on your copyright at the bottom of your page.
All it is going to take is just a super simple little script, but in my opinion it is useful and makes a real difference in how professional a website appears to be.
I am sure you see it often. Small business or personal home pages, very often, but sometimes you even find the website of quiet large companies with outdated copyright notices. - Example 1 - and - Example 2 -
Okay, if you have php installed on your server, this is going to be ridiculously easy, but so useful down the line. I will just cover this in a quick manner:
First off, whatever your html page, you need to save the page as a .php page instead of html. If you have links to the page already, make sure that you update them to .php instead of .html or .htm.
Changing that does nothing else to your page as it is already, it just means that you can now embed the php scripts into the page.
Okay, here is the script -
<?php
$thisYr = date("Y");
if ($thisYr > 2009){
print "<br/>copyright © 2009-" . $thisYr;
}
else {print "<br/>copyright © 2009";}
?>
$thisYr = date("Y");
if ($thisYr > 2009){
print "<br/>copyright © 2009-" . $thisYr;
}
else {print "<br/>copyright © 2009";}
?>
You can simply copy andpaste this into your page where you need it, but I will explain it below:
1. the <?php opens the php code and the ?> closes it. Whatever you write in there is php script.
2. We get the year from the date() function, and by putting the capital 'Y' all we get is the year. So that assigns the current year to the variable called $thisYr. Note that capitalization is important and shouldn't be changed, otherwise you will have trouble with the script.
3. The 'if {} else {}' code blocks are easy to understand. If it is this year, print the one thing, if it isn't print the other. You can change the date to whatever your beginning year is for the copyright.
4. The '©' bit is written like this, but the html page will render it like this '©' It is just the unicode symbols for the little copyright icon.
And that's basically it. You can extend it a little by putting hypertext to the copyright to a link that defines your copyright terms, such as, perhaps, a use and share and backlink or something like that.
If you are creative enough, you may think of other uses for such a simple script. It is one of the wonderful things about these types of scripting languages...small bits of code do really cool things.
Posted by
Vernon
at
4:30 AM
Auto Update Your WebsiteS Copyright Notice Each Year With PHP
2009-10-08T04:30:00-07:00
Vernon
beginner html|copyright notice tutorial|how to php|php|scripting|
Comments

Labels:
beginner html,
copyright notice tutorial,
how to php,
php,
scripting
Monday, October 5, 2009
Non NERD HTML 9 - FORMS
In the last two tutorials we covered lists and tables. Forms follow naturally on from there.
If you have followed along so far, you should be having a lot of fun? We covered the basics of how the page is structured, threw in Favicons for fun, added links and images, and then gave it structure with lists and tables.
So far, though, the only thing that has given your pages any difference from text on a peace of paper has been links, right? Your readers haven't had much to do while they were on your site.
To give them power is in the realm of JavaScript and other scripting languages. The main way that HTML gives your visitors this power of interacting with your website as a program is through forms.
Forms sound rather boring, but in the world of the World Wide Web, forms can let you do a lot of things, and not really appear to be forms.
The problem with forms is that you need scripts to deal with them. It's not tricky, it's just outside the capability of HTML. So I am going to get you started here, but we will visit forms in great detail later when we are working with php.
Form input types:
Some info:
*NOTE* If you don't remember all the others for now, don't worry to much, but try to remember this one. It is the one you are going to use most often.
The 'file' attribute is used when you want your users to load files, such as a photo sharing site like Flickr might have to let users upload their images to the website.
There is another one called 'hidden'. Don't worry about it...it doesn't mean much to us without scripting, so we'll leave it out for now.
Type in the password box...you can see what happens.
The last two are basically for dealing with the form. The Reset button is a courtesy to your users, especially if you have lots of radio or check box buttons. If they messed up, they can 'reset' the form and start over. The 'Submit' button tells the browser that the form is done and can now be sent off.
There is a totally different one. All the above tags fall as attributes of the 'input' tag. But there is one that has it's own tag, and that is 'textarea'
The 'textarea' tag is written totally different. If you look at the code above you will see that two major differences. The one is that the input tags are not closed. Instead we just add a little '/' backslash to the end of them (have a look). The other is that instead of a 'value' attribute, we add the text between the opening and closing tags.
That will be the topic of the next post...the actual structure of html. Just remember it for now.
Okay, lets talk about a couple other aspects. When we make a form, we are going to enclose it in <form> tags. These 'form' tags allow you to say what you want to do with the form.
Now, forms can be really useful, but for now lets just cover the basics.
In the 'form' tag at the top you have two attributes that are typically used with Forms. The first is the 'action' attribute. The action is simply were will we go when the form is submitted. You may simply re-load the current page, but a script in the page detects that there is a variable and accepts this information and does with it whatever it was told to. It may be emailed back to us, it may send them a confirmation email. It may be an automated ordering system. There are many possibilities. A blog post or post on a social media site. Upload pictures. So much of what you do on the web today is done through forms and the scripts behind them.
Aside from the 'action' attribute, there is another that is typically found in the opening 'form' tag, the method. Now again, this isn't going to mean that much to you yet, but will mean a lot when we start scripting. Stick with these tutorials and you will get the power.
Alright, we are almost done with this html tutorial The next section will cover the way the mark up works and include a few new things. After that we will move on to CSS, where we will keep learning some aspects of html as well.
If you have followed along so far, you should be having a lot of fun? We covered the basics of how the page is structured, threw in Favicons for fun, added links and images, and then gave it structure with lists and tables.
So far, though, the only thing that has given your pages any difference from text on a peace of paper has been links, right? Your readers haven't had much to do while they were on your site.
To give them power is in the realm of JavaScript and other scripting languages. The main way that HTML gives your visitors this power of interacting with your website as a program is through forms.
Forms sound rather boring, but in the world of the World Wide Web, forms can let you do a lot of things, and not really appear to be forms.
The problem with forms is that you need scripts to deal with them. It's not tricky, it's just outside the capability of HTML. So I am going to get you started here, but we will visit forms in great detail later when we are working with php.
Form input types:
Name | The mark-up | How it looks |
---|---|---|
Text *NOTE* | <input type="text" name="x" value="Text..." /> | |
Button | <input type="button" name="x" value="button" /> | |
Checkbox | <input type="checkbox" name="x" /> | |
File | <input type="file" name="x" /> | |
Password | <input type="password" name="x" /> | |
Radio | <input type="radio" name="x" /> | |
Reset | <input type="reset" name="x" value="Reset the Form" /> | |
Submit | <input type="text" name="x" value="Text..." /> |
Some info:
*NOTE* If you don't remember all the others for now, don't worry to much, but try to remember this one. It is the one you are going to use most often.
The 'file' attribute is used when you want your users to load files, such as a photo sharing site like Flickr might have to let users upload their images to the website.
There is another one called 'hidden'. Don't worry about it...it doesn't mean much to us without scripting, so we'll leave it out for now.
Type in the password box...you can see what happens.
The last two are basically for dealing with the form. The Reset button is a courtesy to your users, especially if you have lots of radio or check box buttons. If they messed up, they can 'reset' the form and start over. The 'Submit' button tells the browser that the form is done and can now be sent off.
There is a totally different one. All the above tags fall as attributes of the 'input' tag. But there is one that has it's own tag, and that is 'textarea'
Name | The mark-up | How it looks |
---|---|---|
Textarea | <textarea name="x">Type here</textarea> |
The 'textarea' tag is written totally different. If you look at the code above you will see that two major differences. The one is that the input tags are not closed. Instead we just add a little '/' backslash to the end of them (have a look). The other is that instead of a 'value' attribute, we add the text between the opening and closing tags.
That will be the topic of the next post...the actual structure of html. Just remember it for now.
Okay, lets talk about a couple other aspects. When we make a form, we are going to enclose it in <form> tags. These 'form' tags allow you to say what you want to do with the form.
Now, forms can be really useful, but for now lets just cover the basics.
In the 'form' tag at the top you have two attributes that are typically used with Forms. The first is the 'action' attribute. The action is simply were will we go when the form is submitted. You may simply re-load the current page, but a script in the page detects that there is a variable and accepts this information and does with it whatever it was told to. It may be emailed back to us, it may send them a confirmation email. It may be an automated ordering system. There are many possibilities. A blog post or post on a social media site. Upload pictures. So much of what you do on the web today is done through forms and the scripts behind them.
Aside from the 'action' attribute, there is another that is typically found in the opening 'form' tag, the method. Now again, this isn't going to mean that much to you yet, but will mean a lot when we start scripting. Stick with these tutorials and you will get the power.
Alright, we are almost done with this html tutorial The next section will cover the way the mark up works and include a few new things. After that we will move on to CSS, where we will keep learning some aspects of html as well.
Posted by
Vernon
at
1:16 PM
Non NERD HTML 9 - FORMS
2009-10-05T13:16:00-07:00
Vernon
free beginner html|free tutorial|html|html form tutorial|learn html|
Comments

Labels:
free beginner html,
free tutorial,
html,
html form tutorial,
learn html
Subscribe to:
Posts (Atom)